Learn SQL Using PostgreSQL: From Zero to Hero
- Description
- Curriculum
- FAQ
- Reviews
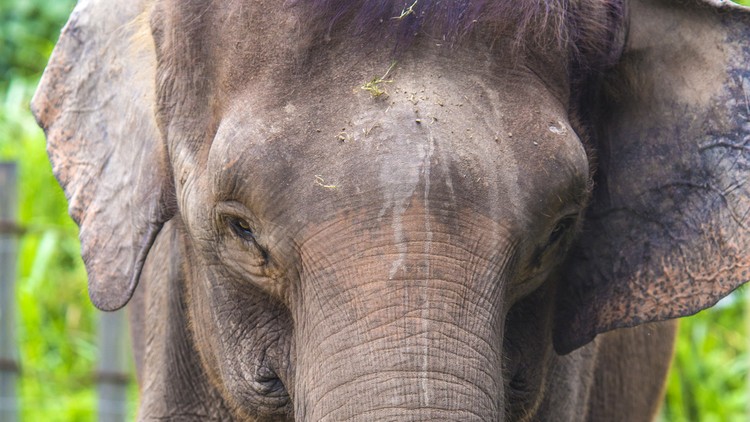
Learning SQL was one of the most valuable skills I learned while building Match. Ā We had one of the largest instances of Microsoft SQL Server in the 90s with millions of records to keep the site running. Ā The better we got at SQL, the better we could make the site work for our users and answers sophisticated questions about our users. Ā
I want to teach you how to use PostgreSQL. Ā We will walk through
-
Basic selection statements
-
Joining multiple tables together
-
Grouping records to get aggregate data
-
Inserting, updating and deleting records
-
Creating tables and indexes
-
Subqueries to create sophisticated reports
-
Table constraints to keep data clean
-
Sequences to create auto incrementing fields
-
CTE – common table expressions that include recursive queries
-
Views to simply accessing complex queries
-
Conditional Expressions for queries
-
Window functions to combine regular queries with aggregate data
-
How to work with date, time and intervals
-
Create SQL Functions to capture complex statements
-
Create PL/pgSQL Functions that allow programming with if/then and loops
-
Triggers
-
Array data types
-
Composite data types
-
Transactions and concurrency control
-
2Installing PostgreSQL on Mac and Windows
How to install PostgreSQL using EnterpriseDB installer
-
3Installing PostgreSQL on Ubuntu
Get up and running with PostgreSQL 11 and pgAdmin 4 on Ubuntu.
-
4Install Northwind Database
How to install Northwind database using pgAdmin using the restore feature and northwind.tar file.
-
5Install Some Additional Databases.
Add 3 more databases to learn from.
-
6Selecting All Data From a Table
How to select all information from a table using simple SELECT statement.
-
7Selecting Specific Fields
How to return specific fields when running a SELECT statement.
-
8Selecting Distinct Values
If you want to find all the unique values in a specific field in table, you use the DISTINCT keyword.
-
9Counting Results
Using COUNT statement to return the number of records.
-
10Combining Fields in SELECT
How to derive information using more than one field.
-
11Practice What You've Learned
Use pagila database to practice your basic SELECT.
-
12What If You Don't Want All Records
Learn about the WHERE clause and how it is used to narrow down the number of records returned.
-
13Searching For Specific Text
How to select records based on matching text fields.
-
14Searching Numeric Fields
How to use WHERE with numeric fields with =, >, >=, <, and <=Ā
-
15Searching Date Fields
How to select records that have date fields.
-
16WHERE Using Logical AND Operator
Using ANDĀ to select records where all conditions must be true.
-
17WHERE Using Logical OR Operator
You can select records where any of the conditions are true using OR operator.
-
18WHERE Using Logical NOT Operator
Reverse the meaning of operator using logical NOTĀ operator.
-
19WHERE Combining AND, OR, and NOT
Using parenthesis to create more complicated queries that combine logical operators.
-
20Using BETWEEN
Using BETWEEN to find values >= and <=.
-
21Using IN
If you have a long list of values the INĀ operator is easier to understand and read.
-
22Practice What You've Learned
Use the usda resource to practice your WHERE clauses.
-
24Connecting With psql
Use psql command line to connect to your local database and run commands.
-
25Eliminate Typing Connection Parameters
Remove inputing connection parameters for command line tools by using environment variables, a .pgpass file, or .pg_service.conf file.
-
26Databases In psql
Learn how to what databases are present and connect to them in psql.
-
27Schemas In psql
How to list the schemas and see the tables in a schema using psql.
-
28ORDER BY
If you need the results to be returned in a specific order, use ORDERĀ BY.
-
29Using MIN and MAX Functions
Find the smallest record with MINĀ and largest with MAX.
-
30Using AVG and SUM
Use these function to find the average value or the sum of all the values.
-
31LIKE to Match Patterns
LIKEĀ allows you to match text patterns for partial matches.
-
32Renaming Columns With Alias
You can change the name of a column with AS syntax.
-
33LIMIT to Control Number of Records Returned
If you only need a certain number of records use LIMITĀ to control.
-
34NULL Values
Nulls are a special value to indicate an unknown. Ā Learn how to use ISĀ NULLĀ and ISĀ NOTĀ NULL to select based on NULLs.
-
35Practice What You've Learned
Practice using AdventureWorks database.
-
36Diagramming Table Relationships
How to map out the tables and relationships in a database with diagrams.
-
37Grabbing Information From Two Tables
How to pull information from 2 different tables in a single statement using JOIN.
-
38Grabbing Information From Multiple Tables
Use multiple JOIN statements to pull together 3 or more tables.
-
39Left Joins
Left joins allow you to pull all records from first table and any matching records from second table.
-
40Right Joins
Right joins allow you to pull matchingĀ records from first table and all records from second table.
-
41Full Joins
Full joins pull all records from both tables.
-
42Self Joins
Connect a table back to itself.
-
43USING To Reduce Typing
Reduce typing with USING instead of ON in joins.
-
44Even Less Typing With NATURAL
NATURAL joins combine tables where fields are named the same in each table.
-
45Practice What You've Learned
Practice joins using the AdventureWorks database.
-
46Group By
GROUP BY allows you to aggregate records and perform an aggregate function like AVG.
-
47Use HAVING to Filter Groups
HAVING clause lets you filter out results of your GROUPĀ BYĀ results.
-
48Grouping Sets
Use GROUPING SETS to group by multiple fields separately in a single query.
-
49Rollup
Using ROLLUPĀ as a shortcut for complex GROUPINGĀ SET
-
50Cube - Rollup On Steroids
CUBE creates all combinations of fields while grouping.
-
54Subquery Using EXISTS
EXISTS subqueries allow you to check a condition in another table as part of the criteria to return a record.
-
55Subquery Using ANY and ALL
Find records that return if any or all of the subquery match the condition.
-
56IN Using Subquery
You can use a subquery with INĀ operator to dynamically build list.
-
57INSERT INTO
You will learn how to insert new data into a table.
-
58UPDATE
Alter existing records using UPDATE statement.
-
59DELETE
Remove data using the DELETEĀ statement.
-
60SELECT INTO
Create a new table based on records returned from select statement.
-
61INSERT INTO SELECT
Insert records into an existing table by selecting from another table.
-
62Returning Data From Update, Delete, and Insert
Using RETURNING to bring back data after INSERT, UPDATE, or DELETE.
-
63What Are Indexes?
Learn what indexes are, what they help with and the drawbacks of too many indexes.
-
64CREATE INDEX
Create indexes on tables that will result in faster searches.
-
65DROP INDEX
Remove an existing index from a table.
-
66How To Kill Runaway Queries
Find running queries using pg_stat_activity table and cancel them with pg_cancel_backend.
-
67Using Explain To See Query Plan
We will create a large table and demonstrate how EXPLAIN works.Ā Then see the difference when an index is added.
-
68Use Analyze To Update Table Statistics
Learn to use EXPLAIN ANALYZE to see actual performance versus the prediction by the query analyzer.Ā Use ANALYZE table_name to update the table statistics.
-
69How Is Query Plan Cost Calculated
Learn how PostgreSQL uses calculates the query plan cost by estimated disk I/O and CPU usage for the query.
-
70Using Indexes On More Than One Field
Learn how to properly use multi-column indexes.Ā
-
71Expression Indexes
Make indexes on modified columns using expression indexes.
-
72Types Of Indexes
Learn about B-Tree, Hash, GIN, GiST, BRIN, and SP-GiST indexes and what situations are best for each type.
-
73Speeding Up Text Matching
Use a GIN index with gin_trgm_ops to speed up text matching in the middle of text for LIKE '%some%' operations.
-
74Design Process Overview
-
75Database Terminology
-
76A Design Process
Learn 7 steps to go through in order to design a database.
-
77Finding Mission Statement And Ojectives
Conduct interviews with employees and management to find out main purpose and tasks the database should support.
-
78Analyzing Current Systems
Learn how to analyze the current paper and database systems in a business and turn into tables and field lists.
-
79Create Table Structure
Take the interviews and information collected in previous step and identify tables and fields that will go in new database.
-
80Establishing Keys
Learn what makes a good primary key and how to find or create one for every table.
-
81Specifying Fields
Document and specify all the fields in database, including descriptions, uniqueness, data type, and length.
-
82Relationships Between Tables
Create a table matrix to map out the relationships between tables. Make an ER diagram to communicate to others what the relationships are.
-
83Business Rules
Learn about different types of business rules and how to document them.
-
84Establish Needed Views
Learn what views are used for and how to map out needed views.
-
85Double Checking Data Integrity
The final step is reviewing data integrity and pulling documentation into a single source.
Social Network